face Chips
Chips can be used to represent small blocks of information. They are most commonly used either for contacts or for tags.
Introduction
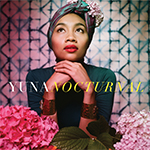
Tag
close
Contacts
To create a contact chip just add an img inside.
<div class="chip">
<img src="img/yuna.jpg" alt="Contact Person">
Jane Doe
</div>
Tags
To create a tag chip just add an close icon inside with the class close
<div class="chip">
Tag
<i class="close material-icons">close</i>
</div>
Javascript Plugin Usage
To add tags, just enter your tag text and press enter. You can delete them by clicking on the close icon or by using your delete button.
Set initial tags.
Use placeholders and override hint texts.
Markup
<div class="chips"></div>
<div class="chips chips-initial"></div>
<div class="chips chips-placeholder"></div>
jQuery Initialization
$('.chips').material_chip();
$('.chips-initial').material_chip({
data: [{
tag: 'Apple',
}, {
tag: 'Microsoft',
}, {
tag: 'Google',
}],
});
$('.chips-placeholder').material_chip({
placeholder: 'Enter a tag',
secondaryPlaceholder: '+Tag',
});
Chip data object
var chip = {
tag: 'chip content',
image: '', //optional
id: 1, //optional
};
jQuery Plugin Options
Option Name | Type | Description |
---|---|---|
data | array | Set the chip data (look at the Chip data object) |
placeholder | string | Set first placeholder when there are no tags. |
secondaryPlaceholder | string | Set second placeholder when adding additional tags. |
Events
Material chips exposes a few events for hooking into chips functionality.
Event | Description |
---|---|
chips.add | this method is triggered when a chip is added. |
chips.delete | this method is triggered when a chip is deleted. |
chips.select | this method is triggered when a chip is selected. |
$('.chips').on('chip.add', function(e, chip){
// you have the added chip here
});
$('.chips').on('chip.delete', function(e, chip){
// you have the deleted chip here
});
$('.chips').on('chip.select', function(e, chip){
// you have the selected chip here
});
Methods
Use these methods to access the chip data.
Parameter | Description |
---|---|
data | It returns the stored data. |
$('.chips-initial').material_chip('data');